WHAT IS DOM? EXPLORING THE RELATIONSHIP BETWEEN DOM AND JAVASCRIPT
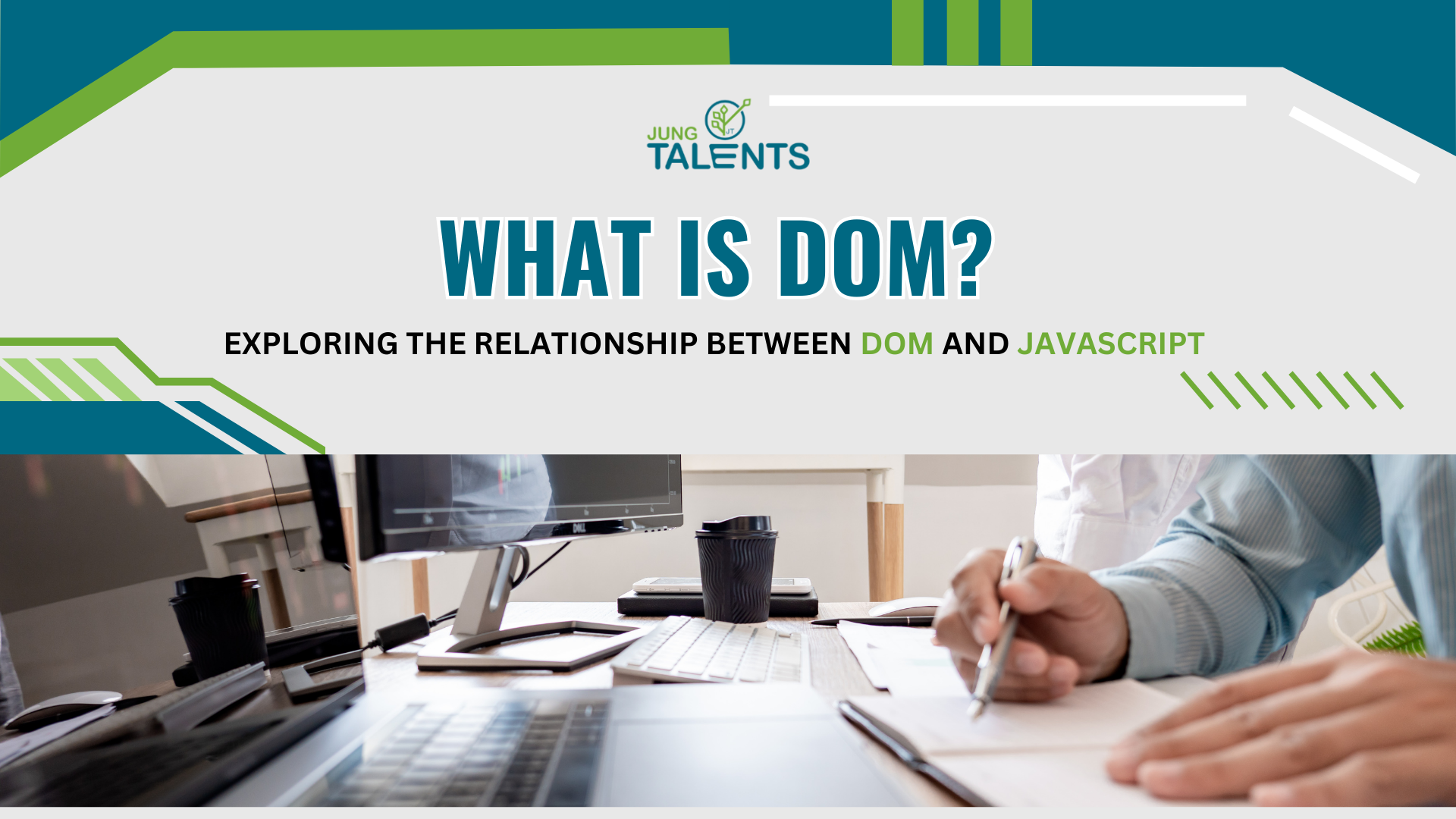
1. What is DOM?
DOM, an abbreviation for Document Object Model, is a representation of the structured objects in a document. It is used to access or manipulate HTML and XML documents in programming languages such as JavaScript, PHP, and more.
According to the World Wide Web Consortium (W3C), this technical standard aims to provide a consistent model for DOM. However, DOM operates independently and relies solely on the descriptive techniques to program specific objects.Within the Document object, we can observe the primary management of HTML tags. The arrangement here branches out into HTML, Body, and finally the Head branches.
In the Head, tags like Style, Title, and more can be found. Conversely, within the Body, any tag is considered an HTML component. Thus, HTML and DOM have an interactive relationship in JavaScript.
2. What is HTML DOM?
HTML DOM can be understood as a tool that facilitates manipulating document data through a model object. Within a document, various structures are defined, such as objects, methods, or attributes, aimed at simplifying access while maintaining the structure. These elements are either objects or owners, with methods to perform actions like deletion, modification, addition, and updates.
Moreover, users can freely add and remove elements as desired to ensure their web content and structure remain updated and refreshed.
3. Details about the DOM Structure Tree
The components constituting the DOM structure are organized into a tree-like branching format, as follows
3.1. Node:
Elements within the DOM are structured as a tree, so the internal model within each small component is called Nodes. These Nodes are classified into various types. However, special attention needs to be given to three main Node types: the root Node, element Node, and text Node.
Specifically:
- Root Node: Also known as the main Node or HTML root document, typically used by the <html> tag.
- Element Node: Used to represent an individual HTML element.
- Text Node: Also known as a Node Text, represents text characters within HTML. When representing a Text Node, it can correspond to a webpage title <title>, a main heading <h1>, or a text paragraph <p>.
3.2. Relationships Between Nodes:
- At the beginning is the Root Node (Document).
- If a Node is not the Root Node, all other Nodes are considered Parent Nodes.
- A Node can contain one or multiple children in a Node, or it might not have any children.
- Nodes within the same Parent Node are referred to as Sibling Nodes.
- Among Sibling Nodes, the first Node is called the eldest child, while the last Node is called the youngest child (FirstChild).
4. Roles of DOM in JavaScript
We can summarize the four basic tasks of DOM in JavaScript as follows:
- Accessing HTML elements.
- Modifying HTML attributes.
- Changing HTML CSS.
- Adding, editing, removing, or creating HTML elements.
5. Categorizing DOM in JavaScript
To facilitate easier and faster handling of HTML, JavaScript divides DOM into different categories, specifically:
- DOM document: Stores the components within the document and webpage.
- DOM element: Mainly responsible for accessing HTML by identifying attributes such as Class, ID, or name within HTML.
- DOM HTML: Supports modifying content or attribute values within HTML.
- DOM CSS: Modifies the CSS formatting of HTML tags.
- DOM Event: Purpose is to assign data, such as onload(), onclick(), and other HTML events.
- DOM Listener: Aids in understanding which events have affected HTML tags.
- DOM Navigation: Used to demonstrate the parent-child relationships within HTML and directly manage operations.
- DOM Node, Nodelist: Used for operations on HTML through objects.
6. Working with DOM in JavaScript
Working with DOM in JavaScript is akin to changing the world. Through DOM, you can manipulate and update content in various ways, such as formatting text, content, Node structure, addition, deletion, modification, and more.
7. Common Attributes and Methods
Let's explore common attributes and methods for working with DOM more easily:
Attributes:
- ID: An attribute of an element often used for direct DOM access.
- ClassName: Similar to ID, used for DOM access, but can be used in multiple elements.
- TagName: HTML tag name.
- InnerHTML: Returns the HTML code within the current element, containing all elements as character strings, including both elements and text Nodes.
- OuterHTML: Returns the HTML code within the current element, effectively outerHTML = tagName + innerHTML.
- TextContent: Returns a character string containing the content of the current text Node.
- Attributes: Set of attributes like ID, Name, Class, Href, Title, etc. Style: Set of formatting for the current element.
- Value: Retrieves the value of a selected element as a variable.
8. Methods:
- GetElementById (ID): Method to access a single element through its ID.
- GetElementsByTagName (Tagname): Accesses all HTML elements with the specified tag name.
- GetElementsByName (Name): Accesses all Nodes with the specified name attribute.
- GetAttribute (Attribute Name): Retrieves the value of an attribute.
- SetAttribute (Attribute Name, value): Modifies the value of an attribute.
- AppendChild (Node): Used to add a child Node to the current Node.
- RemoveChild (Node): Removes a specific Node from the current Node.
In an alternative perspective, the DOM structure tree contains child elements of the DOM. Based on this interaction relationship, DOM can be accessed easily through relationships and positions of HTML elements.
The following relationship attributes represent the interdependence of Nodes:
- ParentNode: Parent Node.
- ChildNodes: Child Nodes.
- FirstChild: First child Node.
- LastChild: Last child Node.
- NextSibling: Sibling Node immediately following.
- PreviousSibling: Sibling Node immediately preceding.
9. Indirect Access in DOM
9.1. Indirect access
Within each Node that forms the DOM's tree structure, six relationship attributes are present to facilitate indirect
Node position: ParentNode: Refers to the parent Node of the current Node, unique to each Node. Therefore, if you need to find the primary source of a Node, you need to concatenate Nodes multiple times, for example: Node.parentNode.parentNode.
- ChildNodes: Refers to the immediate child Nodes of the current Node, creating an array of objects. Note that child Nodes are not differentiated by Node type, so the resulting array may contain different types of Nodes.
- FirstChild: References the first child Node of the current Node, equivalent to calling Node.childNodes [0].
- LastChild: Refers to the last child Node of the current Node, equivalent to calling Node.childNodes [Element.childNodes.length-1].
- NextSibling: Refers to a sibling Node adjacent to the current Node.
- PreviousSibling: Refers to the sibling Node preceding the current Node.
9.2. Direct Access in DOM
Unlike indirect access, using direct access saves time, allowing you to access Nodes in the DOM without an in-depth understanding of the Nodes.
Three methods suitable for direct access include:
1. document.getElementById('id_to_find').
2. document.getElementsByTagName('div').
3.document.getElementsByName('name_to_find').
Conclusion Jung Talents hopes this article provides you with useful information, helping you answer the question "What is DOM?" and understand the relationship between DOM and JavaScript. Jung Talents wishes you success!